Scraping with Python: A Comprehensive Guide to Web Scraping with Python
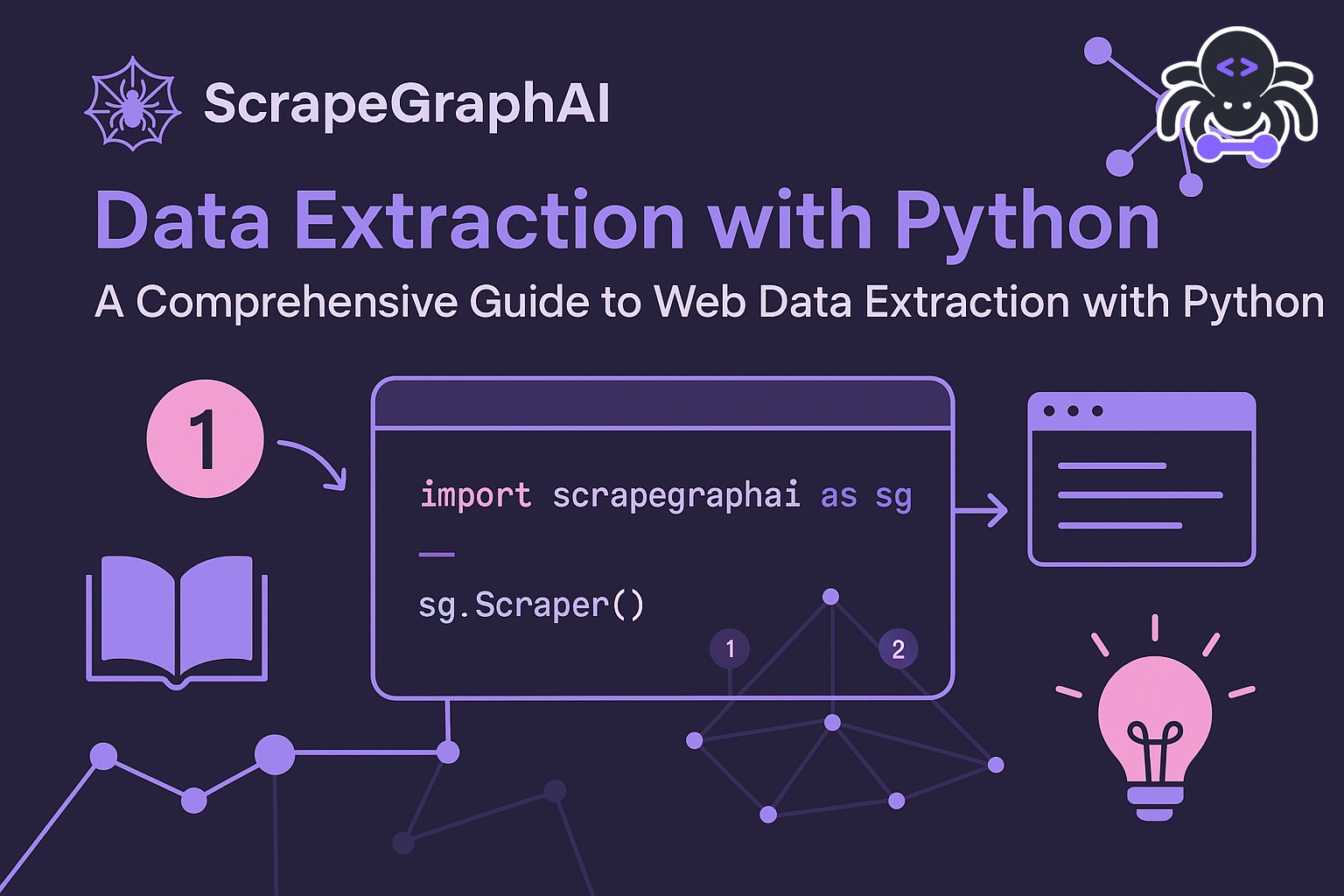
Python has become the go-to language for web scraping due to its simplicity and powerful libraries. Let's explore how to effectively scrape modern websites using Python's best tools and practices.
1. Understanding the Challenge
- Dynamic Content: Modern websites use JavaScript frameworks like React, Vue, and Angular
- API-Driven Data: Content often loads asynchronously through API calls
- Common Obstacles: Infinite scroll, lazy loading, and anti-bot measures
2. Python's Scraping Arsenal
BeautifulSoup4
The classic HTML/XML parser. Perfect for static content and simple scraping tasks.
Selenium
Browser automation powerhouse. Great for JavaScript-heavy sites.
Scrapy
Full-featured scraping framework. Excellent for large-scale projects.
Requests + aiohttp
HTTP clients for making API calls and handling asynchronous requests.
3. Quickstart with BeautifulSoup4
Installation
First, let's set up our environment. You'll need Python and pip installed:
bashpip install beautifulsoup4 requests
Basic Extraction
Here's a simple example using BeautifulSoup4 to extract all headings from a webpage:
pythonimport requests from bs4 import BeautifulSoup def scrape_headings(url): # Send HTTP request response = requests.get(url) response.raise_for_status() # Parse HTML soup = BeautifulSoup(response.text, 'html.parser') # Extract all headings headings = soup.find_all(['h1', 'h2']) return [heading.text.strip() for heading in headings] # Usage url = 'https://example.com' headings = scrape_headings(url) print(headings)
Handle Dynamic Content
For JavaScript-rendered content, we'll need Selenium. Here's how to set it up:
bashpip install selenium webdriver-manager
pythonfrom selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.chrome.options import Options from webdriver_manager.chrome import ChromeDriverManager from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC def scrape_dynamic_content(url): # Setup Chrome options chrome_options = Options() chrome_options.add_argument('--headless') # Initialize the driver driver = webdriver.Chrome( service=Service(ChromeDriverManager().install()), options=chrome_options ) try: # Load the page driver.get(url) # Wait for content to load WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.TAG_NAME, "h1")) ) # Extract content headings = driver.find_elements(By.CSS_SELECTOR, 'h1, h2') return [heading.text for heading in headings] finally: driver.quit() # Usage url = 'https://example.com' headings = scrape_dynamic_content(url) print(headings)
Handle Infinite Scroll
Here's how to handle infinite scroll with Selenium:
pythondef handle_infinite_scroll(driver): last_height = driver.execute_script("return document.body.scrollHeight") while True: # Scroll down driver.execute_script("window.scrollTo(0, document.body.scrollHeight);") # Wait for new content time.sleep(2) # Calculate new scroll height new_height = driver.execute_script("return document.body.scrollHeight") # Break if no more content if new_height == last_height: break last_height = new_height
Bypass Anti-bot Measures
Here's how to make your scraper more human-like:
pythondef setup_stealth_driver(): chrome_options = Options() chrome_options.add_argument('--headless') chrome_options.add_argument('--disable-blink-features=AutomationControlled') chrome_options.add_experimental_option("excludeSwitches", ["enable-automation"]) chrome_options.add_experimental_option('useAutomationExtension', False) driver = webdriver.Chrome( service=Service(ChromeDriverManager().install()), options=chrome_options ) # Modify navigator.webdriver driver.execute_script("Object.defineProperty(navigator, 'webdriver', {get: () => undefined})") return driver
4. Advanced Scraping with Scrapy
Installation
Scrapy is a powerful framework for large-scale scraping:
bashpip install scrapy
Basic Spider
Here's a simple Scrapy spider:
pythonimport scrapy class ExampleSpider(scrapy.Spider): name = 'example' start_urls = ['https://example.com'] def parse(self, response): # Extract headings for heading in response.css('h1, h2'): yield { 'text': heading.css('::text').get(), 'type': heading.css('::attr(tag)').get() } # Follow links for href in response.css('a::attr(href)'): yield response.follow(href, self.parse)
Running the Spider
bashscrapy runspider spider.py -o output.json
5. Best Practices
- Rate Limiting: Use delays between requests
- User Agents: Rotate user agents
- Error Handling: Implement robust error handling
- Proxy Rotation: Use proxy services for large-scale scraping
- Data Storage: Save data incrementally
6. Legal & Ethical Considerations
- Always check robots.txt
- Respect website terms of service
- Implement reasonable request rates
- Store data responsibly
7. Commercial Solutions
- ScrapeGraphAI API-based service with the use of the AI for scraping the web.
- ScrapingBee: API-based scraping service
- ScraperAPI: Proxy rotation and browser automation
- Bright Data: Enterprise-grade scraping infrastructure
Conclusion
Python offers a rich ecosystem for web scraping. Whether you're building a simple scraper with BeautifulSoup4 or a large-scale system with Scrapy, Python has the tools you need. Remember to scrape responsibly and respect website policies.
Ready to simplify your Python web scraping? ScrapeGraphAI empowers you to scrape any website in as little as 5 lines of code, eliminating the usual complexities and headaches. Experience the future of data extraction. Give ScrapeGraphAI a try!
Quick FAQs
BeautifulSoup4 or Selenium?
Use BeautifulSoup4 for static content, Selenium for JavaScript-heavy sites.
How to handle CAPTCHAs?
Consider using commercial services or implementing CAPTCHA solving services.
Best way to store scraped data?
Use databases like PostgreSQL or MongoDB for structured data.
How to scale scraping?
Use Scrapy with distributed crawling or cloud-based solutions.
Is it legal?
Generally yes, if you follow website terms and robots.txt. Always check first.
How to find hidden APIs?
Use browser DevTools > Network tab to monitor XHR/fetch requests.
Did you find this article helpful?
Share it with your network!