101 Web Scraping python
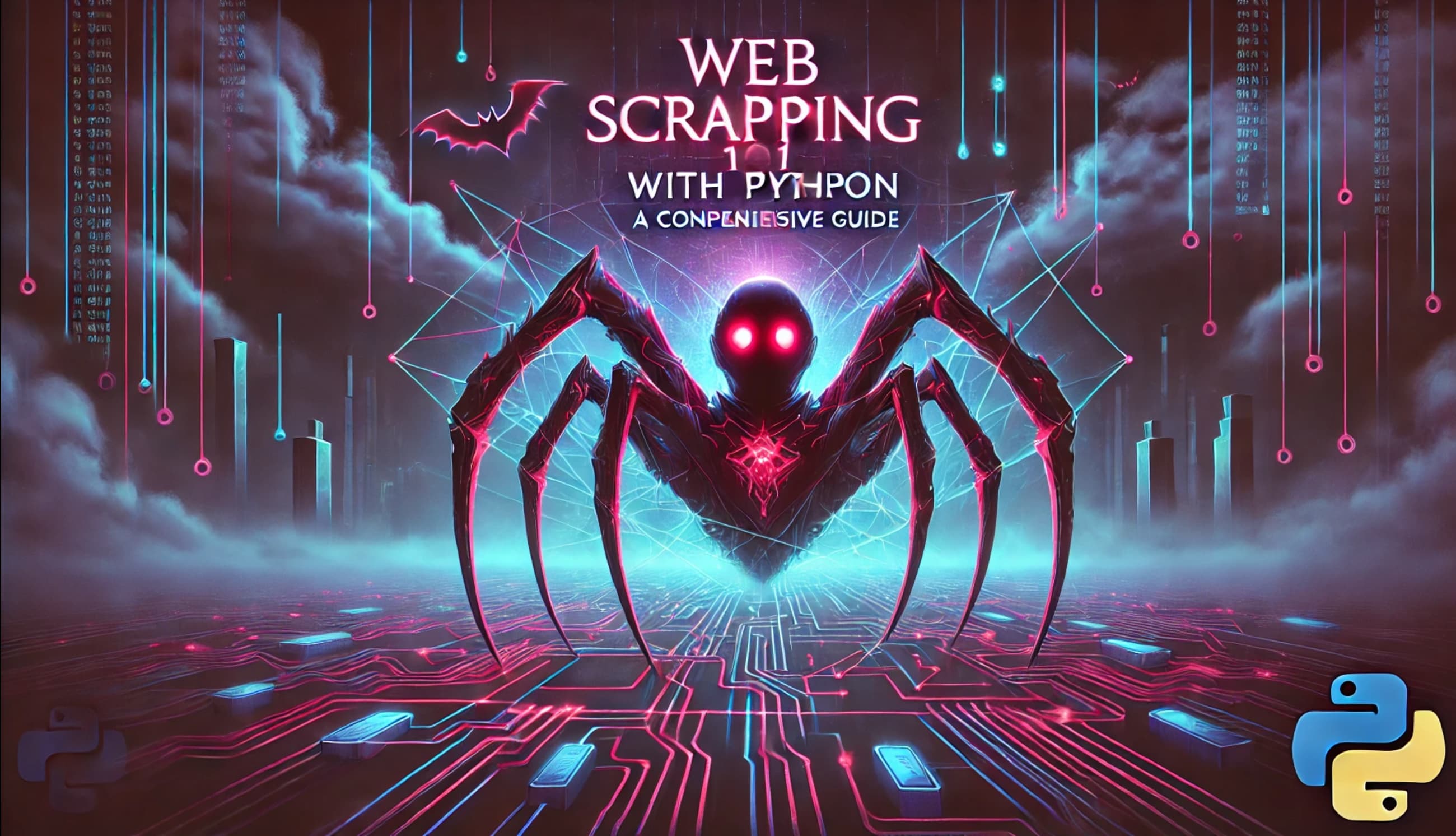
Web Scraping 101 with Python: A Comprehensive Guide
Web scraping is a powerful technique that allows you to extract data from websites automatically. Whether you're looking to monitor product prices, gather research data, or analyze social media trends, Python offers a robust set of tools to help you achieve your goals. In this tutorial, we'll guide you through the essentials of web scraping with Python, providing you with the knowledge to extract data efficiently and responsibly.
Understanding Web Scraping
Web scraping involves programmatically accessing web pages to extract the desired information. It's essential to approach web scraping ethically and responsibly, ensuring compliance with each website's terms of service and legal guidelines.
Setting Up Your Python Environment
Before diving into web scraping, ensure you have Python installed on your system. You can download the latest version from the official Python website. It's advisable to create a virtual environment to manage your project dependencies:
bashpython -m venv scraping-env source scraping-env/bin/activate # On Windows use `scraping-env\Scripts\activate`
Next, install the necessary libraries:
bashpip install requests beautifulsoup4
Inspecting Website Structure
To extract data effectively, you need to understand the structure of the target web page. Use your browser's developer tools (usually accessed by right-clicking on the page and selecting "Inspect") to examine the HTML elements containing the data you wish to scrape.
Using Requests and BeautifulSoup
The requests library allows you to send HTTP requests to retrieve web pages, while BeautifulSoup enables you to parse and navigate the HTML content. Here's a basic example:
pythonimport requests from bs4 import BeautifulSoup url = 'https://example.com' response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') # Extract data data = soup.find_all('tag', class_='class_name') for item in data: print(item.text)
Handling Dynamic Content with Selenium
Some websites load content dynamically using JavaScript, which can make scraping with requests and BeautifulSoup challenging. In such cases, Selenium, a web testing framework, can be used to automate browser interactions and extract dynamic content.
bashpip install selenium
You'll also need to download the appropriate WebDriver for your browser. For example, for Chrome, download ChromeDriver and ensure it's in your system's PATH.
Here's how you can use Selenium to scrape dynamic content:
pythonfrom selenium import webdriver from bs4 import BeautifulSoup # Set up the WebDriver driver = webdriver.Chrome() # Navigate to the page driver.get('https://example.com') # Let the page load completely driver.implicitly_wait(10) # Get page source and parse with BeautifulSoup soup = BeautifulSoup(driver.page_source, 'html.parser') # Extract data data = soup.find_all('tag', class_='class_name') for item in data: print(item.text) # Close the browser driver.quit()
Respecting Robots.txt and Legal Considerations
Before scraping a website, it's crucial to check its robots.txt file to see which parts of the site are allowed to be scraped. Access it by navigating to https://example.com/robots.txt. Always respect the website's terms of service and be aware of legal considerations related to data extraction.
Optimizing and Scaling Your Scraper
As you develop your scraper, consider implementing the following best practices:
- Rate Limiting: Introduce delays between requests to avoid overwhelming the server.
- Error Handling: Implement robust error handling to manage exceptions and retries.
- User-Agent Rotation: Rotate user-agent strings to mimic different browsers and reduce the risk of being blocked.
- Proxy Usage: Use proxies to distribute requests and avoid IP blocking.
For large-scale scraping tasks, consider using frameworks like Scrapy, which offer advanced features for managing requests, handling sessions, and storing data efficiently.
Conclusion
Web scraping with Python is a valuable skill that opens up numerous possibilities for data collection and analysis. By following ethical guidelines and utilizing the right tools and techniques, you can extract meaningful data to support your projects and research.
Remember to always respect website policies, handle data responsibly, and stay informed about legal implications related to web scraping.
Happy scraping!
Did you find this article helpful?
Share it with your network!