Web Scraping with Pydantic: The Ultimate Guide to Structured Data
Learn how to use Pydantic schemas with ScraperGraphAI to create cleaner, more reliable web scraping code with automatic data validation and error handling.
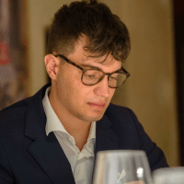
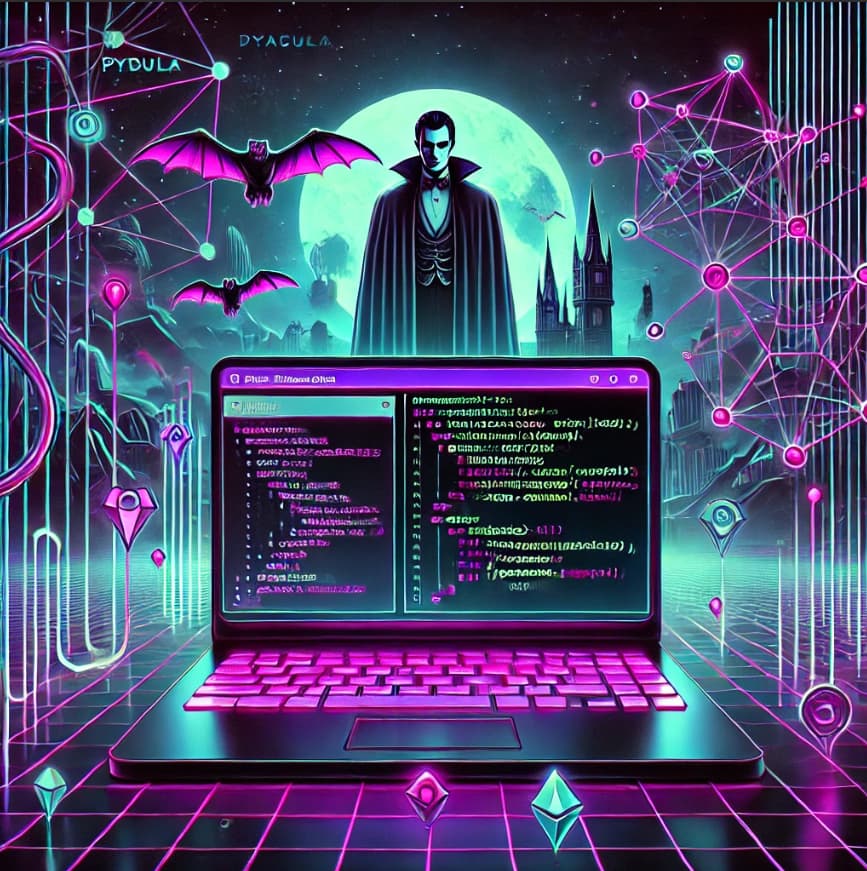
I'm excited to share a simple way to scrape web data using Pydantic schemas. This approach makes your code cleaner and your data more reliable.
Why It Matters
Using Pydantic helps you:
- Keep Data Consistent: Data is automatically checked.
- Catch Errors Early: Problems are found quickly.
- Easily Update Your Code: Clear schemas make changes simple.
How It Works
We combine Pydantic with ScraperGraphAI to define exactly what data we need. Here's an example:
pythonfrom pydantic import BaseModel, Field from scrapegraph_py import Client # Define the schema class WebpageSchema(BaseModel): title: str = Field(description="The title of the webpage") description: str = Field(description="The description of the webpage") summary: str = Field(description="A brief summary of the webpage") # Initialize the client sgai_client = Client(api_key="your-api-key-here") # Make a scraping request with the schema response = sgai_client.smartscraper( website_url="https://example.com", user_prompt="Extract webpage information", output_schema=WebpageSchema, ) print(f"Request ID: {response['request_id']}") print(f"Result: {response['result']}") sgai_client.close()
Example Response
Here's what the extracted data might look like:
json{ "title": "Example Domain", "description": "This domain is for use in illustrative examples in documents.", "summary": "A placeholder website used for documentation and testing purposes." }
Benefits
- Automatic Data Checking: Your data is validated automatically.
- Developer Friendly: Simplifies data parsing and error handling.
- Easy Integration: Works seamlessly with your projects.
Getting Started
- Define Your Schema: Create a Pydantic model for your data.
- Set Up the Client: Initialize ScraperGraphAI with your API key.
- Scrape Data: Use the smartscraper endpoint to get validated data.
Breaking Down the Code
- Schema Definition
We create a Pydantic model that defines the structure of the data we want to extract.
Ready to Scale Your Data Collection?
Join thousands of businesses using ScrapeGrapAI to automate their web scraping needs. Start your journey today with our powerful API.
-
Client Setup
Initialize the ScraperGraphAI client with your API key. -
Making the Request
Use the smartscraper method with your schema to extract structured data. -
Processing Results
The response includes validated data matching your schema.
Frequently Asked Questions
What is Pydantic?
Pydantic is:
- A data validation library
- Type checking tool
- Schema definition system
- Error handling framework
- Data conversion utility
- Documentation generator
How do I define schemas?
Schema definition includes:
- Class creation
- Field definition
- Type specification
- Validation rules
- Documentation
- Testing
What are the benefits?
Benefits include:
- Data validation
- Type safety
- Error handling
- Documentation
- Code clarity
- Maintainability
How do I handle errors?
Error handling includes:
- Validation errors
- Type errors
- Conversion errors
- Custom errors
- Logging
- Recovery
What are the best practices?
Best practices include:
- Clear schemas
- Error handling
- Documentation
- Testing
- Validation
- Maintenance
How do I optimize performance?
Optimization strategies:
- Schema design
- Validation rules
- Error handling
- Resource management
- Monitoring
- Documentation
What about data types?
Data type handling:
- Type checking
- Conversion
- Validation
- Error handling
- Documentation
- Testing
How do I maintain schemas?
Maintenance includes:
- Regular updates
- Documentation
- Testing
- Validation
- Error handling
- Optimization
What about integration?
Integration options:
- API integration
- Database integration
- File handling
- Custom solutions
- Testing
- Documentation
How do I get support?
Support options:
- Documentation
- Community forums
- Support tickets
- Email support
- Social media
- Help center
Conclusion
Using Pydantic with ScraperGraphAI simplifies web scraping and improves data quality. Give it a try to enhance your data extraction process.
Happy scraping!
Related Resources
Want to learn more about structured data extraction? Explore these guides:
- Web Scraping 101 - Master the basics of web scraping
- AI Agent Web Scraping - Learn about AI-powered scraping
- Mastering ScrapeGraphAI - Deep dive into our scraping platform
- Building Intelligent Agents - Create powerful automation agents
- Pre-AI to Post-AI Scraping - See how AI has transformed automation
- Structured Output - Learn about data formatting
- Data Innovation - Discover innovative data methods
- Full Stack Development - Build complete data solutions
- Web Scraping Legality - Understand legal considerations