Real Estate Scraping: The Complete Guide with LangChain
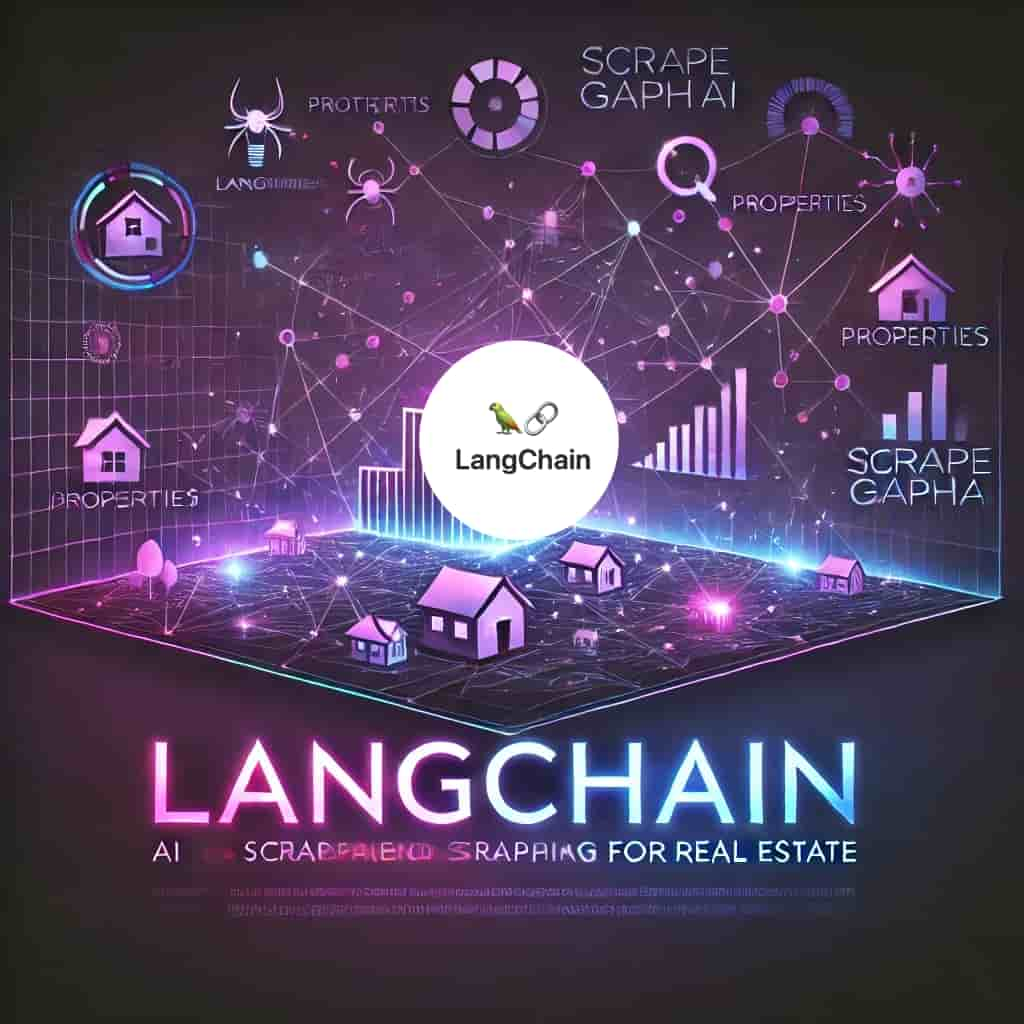
Real Estate Web Scraping: A Comprehensive Guide
Real estate data scraping has become essential for market analysis, property valuation, and investment decisions. This guide will help you understand how to effectively scrape real estate data while following best practices. For beginners, we recommend starting with our web scraping 101 guide before diving into real estate-specific scraping.
Understanding Real Estate Data Sources
Common real estate data sources include:
- Property listing websites
- Real estate marketplaces
- Public records databases
- MLS (Multiple Listing Service) feeds
Learn more about handling dynamic content and structured data extraction for real estate websites.
Setting Up Your Scraping Environment
Before starting your real estate scraping project, ensure you have:
- Python environment (see our web scraping 101 guide)
- Browser automation tools (compare Playwright vs Selenium)
- Data storage solution
- Rate limiting and proxy setup
For advanced setup, check out our guide on scraping without proxies.
Popular Real Estate Platforms
Zillow Scraping
Zillow is one of the largest real estate platforms. Learn specific techniques in our Zillow scraping guide.
Airbnb Data Collection
For vacation rental data, explore our Airbnb scraping guide.
Other Platforms
- Realtor.com
- Redfin
- Trulia
- Local MLS sites
Handling Common Challenges
Dynamic Content
Real estate sites often use JavaScript to load data. Learn how to handle this in our JavaScript scraping guide.
Authentication
Many real estate platforms require authentication. See our guide on handling authentication.
Rate Limiting
Implement proper rate limiting to avoid being blocked. Check our scraping without proxies guide for best practices.
Data Processing and Analysis
Cleaning Real Estate Data
Use tools like Pandas for data cleaning. Learn more in our Pandas guide.
Market Analysis
For advanced analysis, explore our stock analysis guide for relevant techniques.
Legal Considerations
Always respect:
- Website terms of service
- Data privacy laws
- Rate limiting policies
For detailed information, read our web scraping legality guide.
Advanced Techniques
AI-Powered Scraping
Consider using AI for complex real estate data extraction. Learn more in our AI agent web scraping guide.
Multi-Agent Systems
For large-scale real estate data collection, explore multi-agent systems.
Real-World Applications
Real estate scraping can be used for:
- Market trend analysis
- Property valuation
- Investment opportunities
- Rental market research
Best Practices
-
Data Quality
- Validate property information
- Handle missing data
- Regular data updates
-
Performance
- Implement caching
- Use efficient data structures
- Optimize storage
-
Maintenance
- Monitor site changes
- Update selectors
- Handle errors gracefully
For more optimization techniques, check our structured data extraction guide.
Conclusion
Real estate web scraping is a powerful tool for market analysis and investment decisions. By following best practices and using the right tools, you can gather valuable insights from real estate data.
For those looking to expand their knowledge, explore our guides on:
Remember to check out our web scraping tutorials for more in-depth guides and best practices.
In today's data-driven world, having high-quality datasets is essential. However, collecting data can often be challenging, expensive, and time-consuming. With tools like ScrapeGraphAI and ๐ LangChain, creating datasets becomes much simpler and faster. In this post, we'll show you how to use these tools to collect data intelligently.
Why Use ScrapeGraphAI and LangChain?
ScrapeGraphAI and LangChain are a powerful combination for collecting and organizing data efficiently. ScrapeGraphAI uses advanced AI to handle even the most complex websites, structuring data clearly while ensuring scalability and cleanliness. LangChain complements this by enabling the creation of intelligent AI-powered programs that can maximize the value of collected data. Together, they simplify building robust software solutions that depend on high-quality datasets.
Ready to Scale Your Data Collection?
Join thousands of businesses using ScrapeGrapAI to automate their web scraping needs. Start your journey today with our powerful API.
Setting Up Your Environment
Before you begin, make sure you have:
- Python (Version 3.8 or later)
- LangChain-ScrapeGraph: Install with pip install langchain-scrapegraph
Building Your Dataset: A Step-by-Step Guide
Step 1: Define Your Data Requirements
Think about the websites or APIs that contain the data you're looking for. For example, you might want to collect information about houses for sale from sites like Zillow. Consider what specific data points you need:
- Property details (price, bedrooms, bathrooms)
- Location information
- Agent details
- Additional features and amenities
Step 2: Configure ScrapeGraphAI
First, you'll need to set up your environment and authenticate:
pythonimport os from getpass import getpass # Check if the API key is already set sgai_api_key = os.getenv("SGAI_API_KEY") if sgai_api_key: print("SGAI_API_KEY found.") else: print("SGAI_API_KEY not found.") sgai_api_key = getpass("Enter your SGAI_API_KEY: ").strip() if sgai_api_key: os.environ["SGAI_API_KEY"] = sgai_api_key print("SGAI_API_KEY set.")
Next, define your data schema to ensure structured output:
pythonfrom pydantic import BaseModel, Field from typing import List, Optional class HouseListingSchema(BaseModel): price: int = Field(description="Price of the house in USD") bedrooms: int = Field(description="Number of bedrooms") bathrooms: int = Field(description="Number of bathrooms") square_feet: int = Field(description="Total square footage") address: str = Field(description="Address of the house") city: str = Field(description="City where the house is located") state: str = Field(description="State where the house is located") zip_code: str = Field(description="ZIP code of the house") tags: List[str] = Field(description="Tags like 'New construction' or 'Large garage'") agent_name: str = Field(description="Name of the agent") agency: str = Field(description="Real estate agency") class HousesListingsSchema(BaseModel): houses: List[HouseListingSchema] = Field(description="List of house listings")
Step 3: Implement the Scraper
Now you can create your scraper instance and start collecting data:
pythonfrom langchain_scrapegraph.tools import SmartScraperTool tool = SmartScraperTool() def extract_house_listings(url: str, api_key: str): response = tool.scrapegraph_smartscraper( prompt="Extract information about the houses visible on the page", url=url, api_key=api_key, schema=HousesListingsSchema, ) return response["result"] url = "https://www.homes.com/san-francisco-ca/" house_listings = extract_house_listings(url, sgai_api_key)
Step 4: Process and Store the Data
Finally, save your data in a format that suits your needs:
pythonimport json import pandas as pd # Save as JSON for flexibility with open("houses.json", "w") as f: json.dump(house_listings, f, indent=2) # Create a DataFrame for analysis df = pd.DataFrame(house_listings["houses"]) df.to_csv("houses_for_sale.csv", index=False)
Key Applications and Use Cases
-
Real Estate Market Analysis
- Track property prices and trends
- Analyze market dynamics
- Monitor competitor listings
-
E-Commerce Intelligence
- Price optimization
- Competitor monitoring
- Product trend analysis
-
Healthcare Data Collection
- Medical research aggregation
- Healthcare provider information
- Treatment cost analysis
-
Financial Market Research
- Investment opportunities
- Market sentiment analysis
- Economic indicators tracking
For more examples and detailed use cases, check out our ๐ ScrapeGraphAI Cookbook which contains ready-to-use recipes for various scraping scenarios.
Best Practices for Efficient Scraping
When using ScrapeGraphAI for data collection, keep these tips in mind:
- Respect Rate Limits: Always implement appropriate delays between requests
- Validate Data: Always verify the quality and completeness of collected data
Frequently Asked Questions
What data can I extract from real estate websites?
You can extract various data points including:
- Property prices and details
- Location information
- Agent details
- Property features and amenities
- Market trends
- Historical data
How do I handle rate limiting?
Best practices include:
- Implementing appropriate delays
- Using proxy rotation
- Respecting website terms of service
- Monitoring request patterns
- Managing concurrent requests
- Following ethical guidelines
What are the common challenges in real estate scraping?
Common challenges include:
- Dynamic content loading
- Anti-bot measures
- Data validation
- Rate limiting
- Website structure changes
- Data consistency
How do I ensure data accuracy?
Ensure accuracy through:
- Regular validation checks
- Cross-referencing sources
- Data cleaning processes
- Error handling
- Quality assurance
- Automated testing
What are the best practices for real estate data collection?
Best practices include:
- Respecting website terms
- Implementing proper delays
- Using reliable proxies
- Validating data
- Maintaining documentation
- Regular monitoring
How can I scale my real estate scraping operations?
Scaling strategies include:
- Distributed scraping
- Load balancing
- Resource optimization
- Parallel processing
- Efficient data storage
- Monitoring systems
What legal considerations should I be aware of?
Important considerations:
- Website terms of service
- Data privacy laws
- Copyright issues
- Usage restrictions
- Compliance requirements
- Ethical guidelines
How do I handle different property types?
Considerations include:
- Different data structures
- Varying information fields
- Special requirements
- Format standardization
- Data categorization
- Validation rules
What about data storage and management?
Storage considerations:
- Database selection
- Data organization
- Backup strategies
- Access control
- Data retention
- Security measures
How do I keep my scraping solution up to date?
Maintenance includes:
- Regular monitoring
- Code updates
- Structure adaptation
- Performance optimization
- Error handling
- Documentation updates
Conclusion
The combination of ScrapeGraphAI and ๐ LangChain provides a powerful solution for modern data collection needs. Whether you're analyzing real estate markets, tracking e-commerce trends, or gathering research data, these tools make the process efficient and reliable.
Ready to Scale Your Data Collection?
Join thousands of businesses using ScrapeGrapAI to automate their web scraping needs. Start your journey today with our powerful API.
Did you find this article helpful?
Share it with your network!