Playwright vs Selenium: Choosing the Right Tool for Web Scraping
·2 min read min read·Tips & Resources
Share:
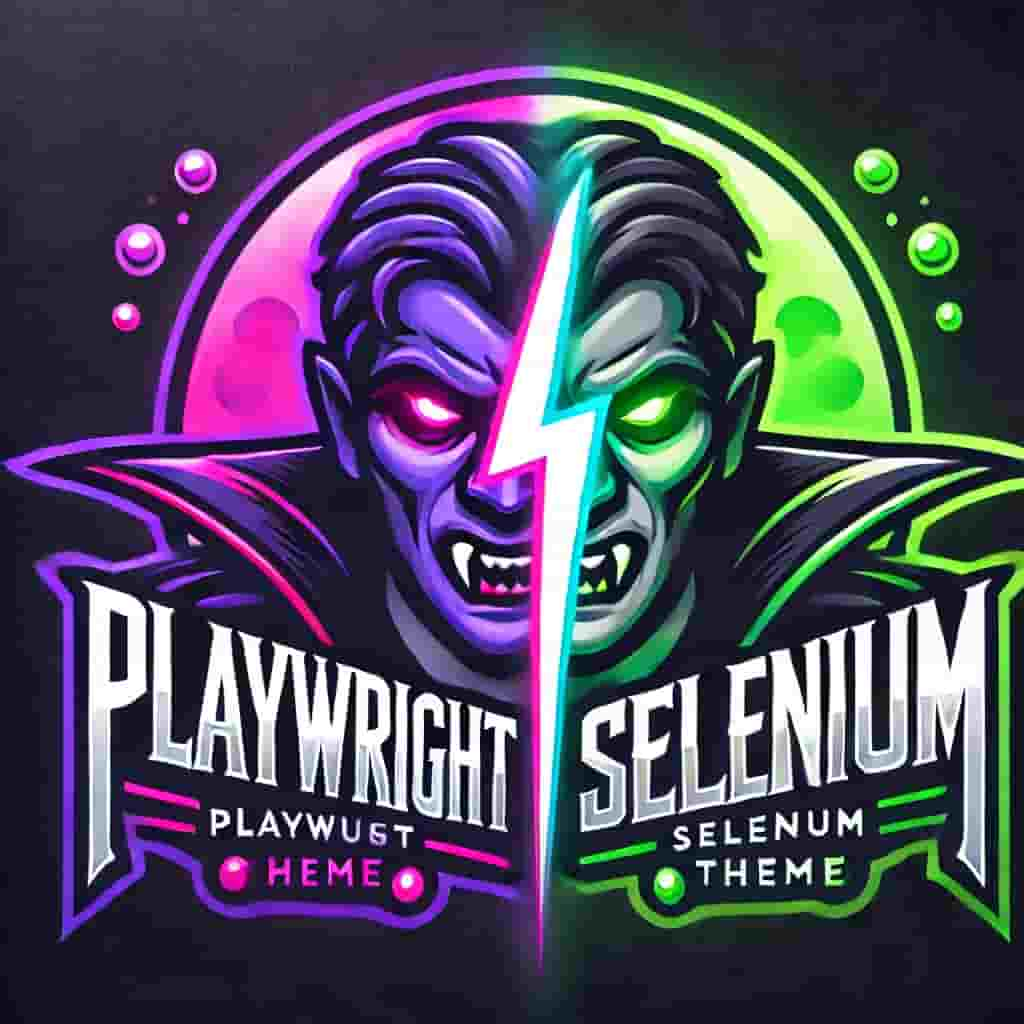
When it comes to web scraping, choosing the right automation tool is crucial. In this comprehensive comparison, we'll explore two popular options: Playwright and Selenium. We'll help you make an informed decision based on your specific needs.
Key Differences
1. Architecture
- Playwright: Modern, built for today's web, supports multiple browser engines
- Selenium: Mature, widely adopted, WebDriver protocol-based
2. Performance
- Playwright: Faster execution, better resource management
- Selenium: More resource-intensive, slower execution
3. Features
- Playwright:
- Auto-wait capabilities
- Network interception
- Multiple tabs/contexts
- Mobile emulation
- Selenium:
- Extensive language support
- Large community
- More third-party tools
- Grid support for scaling
Code Comparison
Basic Navigation
Playwright:
pythonfrom playwright.sync_api import sync_playwright with sync_playwright() as p: browser = p.chromium.launch() page = browser.new_page() page.goto('https://example.com') page.wait_for_selector('.content') title = page.title() browser.close()
Selenium:
pythonfrom selenium import webdriver from selenium.webdriver.common.by import By from selenium.webdriver.support.ui import WebDriverWait from selenium.webdriver.support import expected_conditions as EC driver = webdriver.Chrome() driver.get('https://example.com') element = WebDriverWait(driver, 10).until( EC.presence_of_element_located((By.CLASS_NAME, "content")) ) title = driver.title driver.quit()
When to Choose Each Tool
Choose Playwright When:
- Modern web application testing is needed
- Performance is crucial
- Network manipulation is required
- Multiple browser contexts are needed
Choose Selenium When:
- Legacy application support is required
- Language flexibility is important
- Grid infrastructure is needed
- Extensive community support is valued
Best Practices
-
Error Handling
- Implement robust try-except blocks
- Use explicit waits over implicit waits
- Handle timeouts gracefully
-
Resource Management
- Always close browsers/drivers
- Implement proper cleanup
- Monitor memory usage
-
Performance Optimization
- Use headless mode when possible
- Minimize wait times
- Batch operations when feasible
Conclusion
Both Playwright and Selenium have their strengths. Playwright excels in modern web automation with better performance, while Selenium offers mature ecosystem and broader language support. Choose based on your specific requirements and use case.
Did you find this article helpful?
Share it with your network!
Share: