Scraping JavaScript-Rendered Websites: Tools, Tips, and Tactics
Learn how to scrape JavaScript-rendered websites using ScrapeGraphAI's Smart Scraper. This guide covers everything from basic scraping concepts to implementing advanced data extraction techniques for customer feedback analysis.
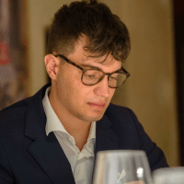
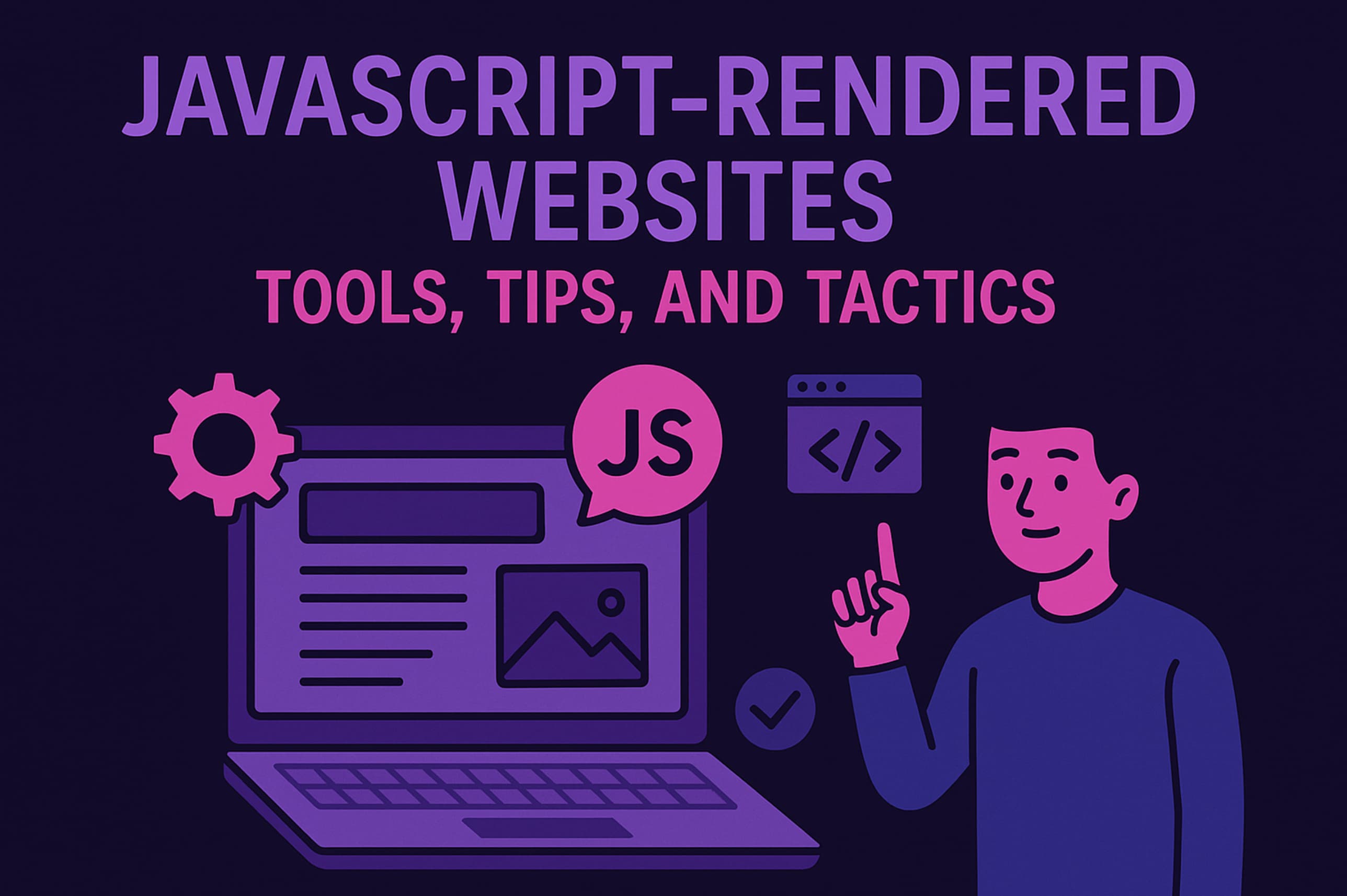
Web Scraping with JavaScript: A Complete Guide
JavaScript has become an essential tool for modern web scraping, especially when dealing with dynamic websites. This guide will help you master JavaScript-based web scraping techniques. For beginners, we recommend starting with our web scraping 101 guide before diving into JavaScript-specific scraping.
Why Use JavaScript for Scraping?
JavaScript is particularly useful for:
- Handling dynamic content
- Managing browser automation
- Processing client-side rendered pages
- Interacting with modern web applications
Learn more about browser automation tools and handling dynamic content.
Setting Up Your Environment
Required Tools
-
Node.js Environment
- Install Node.js from nodejs.org
- Set up npm for package management
-
Browser Automation Tools
- Playwright (recommended)
- Puppeteer
- Selenium
Compare these tools in our Playwright vs Selenium guide.
Basic JavaScript Scraping
Using Playwright
javascriptconst { chromium } = require('playwright'); async function scrapeWebsite() { const browser = await chromium.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); // Extract data const data = await page.evaluate(() => { return document.querySelector('.content').innerText; }); await browser.close(); return data; }
For more advanced Playwright examples, check our browser automation guide.
Using Puppeteer
javascriptconst puppeteer = require('puppeteer'); async function scrapeWithPuppeteer() { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); const data = await page.evaluate(() => { return Array.from(document.querySelectorAll('.item')) .map(item => item.innerText); }); await browser.close(); return data; }
Handling Common Scenarios
Dynamic Content Loading
Learn how to handle:
- Infinite scroll
- Lazy loading
- AJAX requests
- WebSocket connections
See our guide on structured data extraction for more details.
Authentication
Handle various authentication methods:
- Login forms
- OAuth
- API keys
- Session management
Check our mastering ScrapeGraphAI endpoint guide for advanced authentication techniques.
Rate Limiting and Proxies
Implement:
- Request throttling
- Proxy rotation
- IP management
- Session handling
Learn more in our scraping without proxies guide.
Advanced Techniques
AI-Powered Scraping
Consider using AI for complex scraping tasks:
- Pattern recognition
- Content extraction
- Navigation automation
- Error handling
Explore our AI agent web scraping guide for more information.
Multi-Agent Systems
For large-scale scraping:
- Task distribution
- Parallel processing
- Error recovery
- Data validation
Learn about multi-agent systems in our dedicated guide.
Best Practices
-
Error Handling
- Implement retry mechanisms
- Handle timeouts
- Manage browser crashes
- Log errors effectively
-
Performance Optimization
- Use headless mode
- Implement caching
- Optimize selectors
- Manage memory usage
-
Maintenance
- Monitor site changes
- Update selectors
- Handle updates
- Maintain documentation
For more optimization techniques, check our structured data extraction guide.
Real-World Applications
JavaScript scraping can be used for:
Conclusion
JavaScript web scraping is a powerful approach for handling modern websites. By following best practices and using the right tools, you can create robust and efficient scraping solutions.
For those looking to expand their knowledge, explore our guides on:
Remember to check out our web scraping tutorials for more in-depth guides and best practices.
1. What's the Real Issue?
- Client-side rendering: Frameworks like React, Vue, and Angular build the DOM in the browser after the initial HTML loads.
- Asynchronous data: Content often arrives via API calls in the background. Timing is everything.
- Common headaches: Infinite scroll, lazy-loaded elements, bot protection (CAPTCHA, CSRF tokens)—scraping isn't always easy.
2. Your Weaponry
-
Puppeteer (Node.js) A simple API for controlling Chrome/Chromium headless browsers. You can open pages, click buttons, extract DOM elements.
-
Playwright Very similar to Puppeteer, but works with Chromium, Firefox, and WebKit—great for cross-browser scraping.
-
Selenium The OG tool. It supports multiple languages and browsers. Slightly heavier, but rock solid.
Ready to Scale Your Data Collection?
Join thousands of businesses using ScrapeGrapAI to automate their web scraping needs. Start your journey today with our powerful API.
- No-Code Solutions Don't want to write code? Use Octoparse, ParseHub, or Apify for drag-and-drop scraping workflows.
3. Quickstart with Puppeteer
Install Node & Puppeteer
First, let's set up our development environment. You'll need Node.js installed on your system. Then, create a new project and install Puppeteer:
bashnpm init -y npm install puppeteer
Basic Extraction
Now that we have Puppeteer installed, let's create our first scraping script. This example shows how to launch a headless browser, navigate to a page, and extract all headings. The script waits for the network to be idle, ensuring all content is loaded before extracting data:
tsimport puppeteer from 'puppeteer'; (async () => { const browser = await puppeteer.launch({ headless: true }); const page = await browser.newPage(); await page.goto('https://example.com', { waitUntil: 'networkidle0' }); const data = await page.evaluate(() => { return Array.from(document.querySelectorAll('h1, h2')).map(el => el.innerText); }); console.log(data); await browser.close(); })();
Handle Infinite Scroll
Many modern websites use infinite scroll to load content as you scroll down. Here's how to handle this pattern. The code scrolls to the bottom of the page, waits for new content to load, and repeats until no more content appears. The 2-second delay between scrolls helps prevent overwhelming the server:
tslet previousHeight; do { previousHeight = await page.evaluate(() => document.body.scrollHeight); await page.evaluate(() => window.scrollTo(0, document.body.scrollHeight)); await page.waitForTimeout(2000); } while (await page.evaluate(() => document.body.scrollHeight) > previousHeight);
Bypass Anti-bot Measures
Websites often implement measures to detect and block bots. This code helps your scraper look more like a real browser by setting a realistic user agent and adding random delays between actions. The random delay between 1-3 seconds makes your scraping behavior appear more human-like:
tsawait page.setUserAgent('Mozilla/5.0 (Windows NT 10.0; Win64; x64)...'); await page.waitForTimeout(Math.random() * 2000 + 1000);
4. Quickstart with Playwright
Install Playwright
Playwright offers a more modern approach to browser automation. It's installed as a development dependency since it's typically used in testing and automation scripts:
bashnpm install -D playwright
Basic Extraction
Playwright provides a more concise API compared to Puppeteer. This example shows how to extract headings using Playwright's built-in evaluation methods. Notice how we don't need to manually create an array from the NodeList:
tsimport { chromium } from 'playwright'; (async () => { const browser = await chromium.launch(); const page = await browser.newPage(); await page.goto('https://example.com'); const titles = await page.$$eval('h1, h2', els => els.map(el => el.textContent)); console.log(titles); await browser.close(); })();
Scroll to Load More
Playwright offers a more elegant solution for handling infinite scroll. This implementation uses a Promise-based approach with a timer, providing smoother scrolling and better control over the scroll speed. The 300ms interval between scrolls can be adjusted based on the website's loading speed:
tsawait page.evaluate(async () => { await new Promise(resolve => { let totalHeight = 0; const distance = 100; const timer = setInterval(() => { window.scrollBy(0, distance); totalHeight += distance; if (totalHeight >= document.body.scrollHeight) { clearInterval(timer); resolve(); } }, 300); }); });
5. Quickstart with Selenium (JavaScript Example)
Install
Selenium is the most established browser automation tool. It requires both the Selenium WebDriver and a browser-specific driver. For Chrome, we'll use chromedriver:
bashnpm install selenium-webdriver chromedriver
Basic Usage
Selenium's approach is more verbose but offers great flexibility. This example demonstrates how to set up a WebDriver instance, navigate to a page, and extract elements using CSS selectors. The try-finally block ensures the browser is properly closed even if an error occurs:
tsimport { Builder, By } from 'selenium-webdriver'; (async function example() { let driver = await new Builder().forBrowser('chrome').build(); try { await driver.get('https://example.com'); let headers = await driver.findElements(By.css('h1, h2')); for (let header of headers) { console.log(await header.getText()); } } finally { await driver.quit(); } })();
6. SEO Tips to Make Your Post Fly
- Title & H1: Put the main keyword up front. Example: "Scrape JavaScript Sites Easily: A Practical Guide."
- Clean URLs: Use short paths like "/scrape-javascript-sites-easily/", no dates.
- Meta Description: Scrape JavaScript sites easily with Puppeteer, Playwright, or Selenium. A complete, practical guide in under 155 characters.
- Headings: Use clear, structured H2/H3s that reflect each section.
- Featured Snippet Friendly: Use bullet points, numbered steps, and code blocks for better chances of ranking.
7. Legal & Ethical Considerations
- Always read the site's Terms of Service.
- Respect "robots.txt" (even if it's not legally binding).
- Avoid overloading servers with aggressive scraping rates.
Conclusion & Next Steps
You now have all the tools to scrape JavaScript sites easily. Launch a Puppeteer script in minutes, experiment with pagination, anti-bot tactics, and multiple frameworks. And don't forget—always scrape responsibly.
Quick FAQs
Puppeteer or Playwright?
If you only need Chrome, go with Puppeteer. For cross-browser support, use Playwright.
Do I always need a headless browser?
Only if the site uses JavaScript to render the content. If not, HTTP requests may be enough.
How do I scale?
Use Docker/Kubernetes or commercial services like Apify.
Is it legal?
Generally yes, if you're accessing public data responsibly. But always check the site's ToS.
How do I find hidden APIs?
Use browser DevTools > Network tab to sniff out "XHR" or "fetch" requests.
Related Resources
Want to learn more about JavaScript web scraping? Explore these guides:
- Web Scraping 101 - Master the basics of web scraping
- AI Agent Web Scraping - Learn about AI-powered scraping
- Mastering ScrapeGraphAI - Deep dive into our scraping platform
- Building Intelligent Agents - Create powerful automation agents
- Pre-AI to Post-AI Scraping - See how AI has transformed automation
- Structured Output - Learn about data formatting
- Data Innovation - Discover innovative data methods
- Full Stack Development - Build complete data solutions
- Web Scraping Legality - Understand legal considerations
These resources will help you master JavaScript web scraping while building powerful solutions.
export const metadata = { // ... existing code ...