Building Python MCP Servers: A Step-by-Step Guide with FastMCP
Learn how to build a Python MCP server using FastMCP and integrate it with Zapier MCP to automate complex workflows.
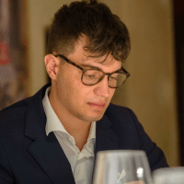
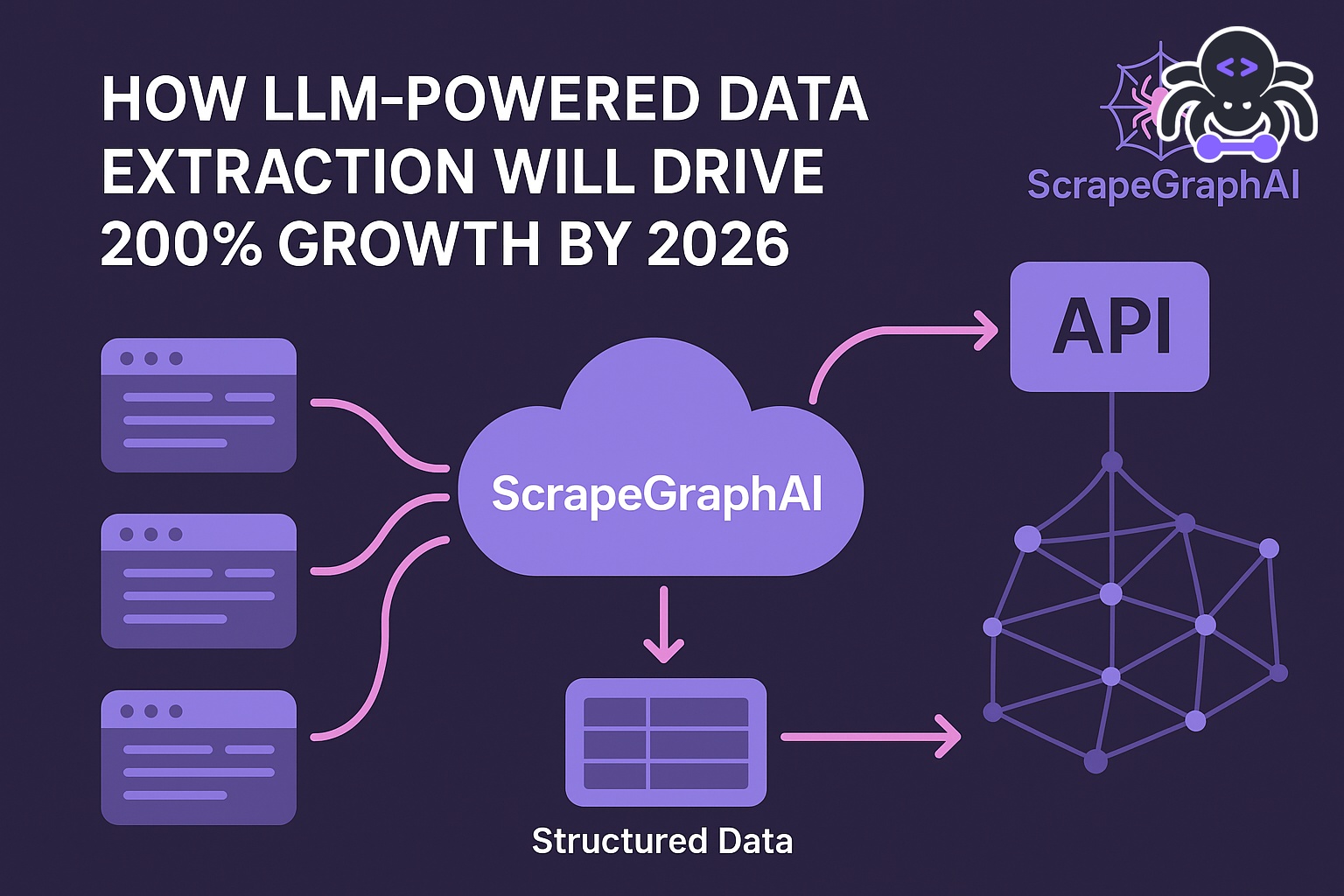
This tutorial shows you how to create a custom Model Context Protocol (MCP) server in Python using FastMCP, then connect it to Zapier MCP to let your AI agents perform real-world tasks across thousands of apps.
Why Combine FastMCP and Zapier MCP?
- FastMCP: A Python SDK for MCP servers, inspired by FastAPI, enabling rapid prototyping of MCP endpoints.
- Zapier MCP: A hosted MCP service offering access to 7,000+ apps and 30,000+ actions via a single standard MCP URL.
- MCP Standard: Ensures secure, structured communication between AI models and external tools.
Prerequisites
bashpip install fastmcp scrapegraph_py
Step 1: Build Your FastMCP Server
Create a file called server.py:
pythonfrom fastmcp.server import Server # Initialize your FastMCP server server = Server() @server.listener() def on_login(event): player = event.player player.send_message("Welcome to your custom MCP server!") @server.listener() def on_custom_action(event): # Example: Receive an MCP request to trigger a Zapier automation action_payload = event.payload # Forward payload to Zapier MCP endpoint... # (We'll set this up in Step 3) event.respond({"status": "forwarded", "payload": action_payload}) # Run the server if __name__ == "__main__": server.run(host="0.0.0.0", port=8080)
Step 2: Define Your MCP Schema
(Optional) Use Pydantic to enforce structure on incoming requests:
pythonfrom pydantic import BaseModel, Field class ZapierAction(BaseModel): app: str = Field(description="Zapier app key, e.g., 'slack'") action: str = Field(description="Action name, e.g., 'send_message'") data: dict = Field(description="Payload data for the action")
Step 3: Connect to Zapier MCP
Replace the placeholder in on_custom_action to forward requests:
pythonimport requests ZAPIER_MCP_URL = "https://api.zapier.com/mcp" # Your Zapier MCP endpoint @server.listener() def on_custom_action(event): action = ZapierAction(**event.payload) response = requests.post( ZAPIER_MCP_URL, json={ "app": action.app, "action": action.action, "data": action.data }, headers={"Authorization": "Bearer your-zapier-api-key"} ) return response.json()
Example Usage
Send a request from your MCP client:
pythonfrom mcp.client.fastmcp import MCPClient
Ready to Scale Your Data Collection?
Join thousands of businesses using ScrapeGrapAI to automate their web scraping needs. Start your journey today with our powerful API.
client = MCPClient(server_url="http://localhost:8080") result = client.call({ "method": "on_custom_action", "params": { "app": "slack", "action": "send_message", "data": { "channel": "#general", "text": "Hello from FastMCP!" } } }) print(result)
text## Benefits - Full Control: Host your own MCP server logic. - Broad Connectivity: Leverage Zapier's ecosystem of apps and actions. - Standards-Compliant: Follow the MCP spec for secure, structured tool calls. ## Next Steps 1. Enhance your FastMCP server with more event handlers. 2. Explore Zapier templates to automate complex workflows. 3. Integrate additional MCP clients or hosts (Cursor, Claude Desktop, etc.). Start building powerful AI-driven automations today by combining FastMCP with Zapier MCP! 🚀 ## Frequently Asked Questions ### What is Model Context Protocol? MCP features: - Standardized communication - Tool integration - Context management - Secure messaging - Event handling - State management ### How does FastMCP work? FastMCP provides: - Easy setup - Event handling - State management - Tool integration - Error handling - Secure communication ### What can I build with MCP? Applications include: - AI agents - Tool integrations - Automation systems - Event handlers - State managers - Communication systems ### What are the key benefits? Benefits include: - Standardization - Easy integration - Secure messaging - Event handling - State management - Tool connectivity ### What tools are needed? Essential tools: - FastMCP - Python environment - Development tools - Integration APIs - Error handling - Monitoring systems ### How do I ensure reliability? Reliability measures: - Error handling - State validation - Event monitoring - System checks - Logging - Testing ### What are common challenges? Challenges include: - Integration complexity - State management - Error handling - Scale requirements - Performance tuning - Resource management ### How do I optimize performance? Optimization strategies: - Efficient routing - State caching - Resource allocation - Load balancing - Error handling - Performance monitoring ### What security measures are important? Security includes: - Message encryption - State protection - Access control - Error handling - Audit logging - Secure communication ### How do I maintain MCP systems? Maintenance includes: - Regular updates - Performance checks - Error monitoring - System optimization - Documentation - Staff training ### What are the costs involved? Cost considerations: - Development time - Infrastructure - Maintenance - Updates - Support - Training ### How do I scale operations? Scaling strategies: - Load distribution - Resource optimization - System monitoring - Performance tuning - Capacity planning - Infrastructure updates ### What skills are needed? Required skills: - Python programming - API integration - Error handling - System design - Performance tuning - Protocol understanding ### How do I handle errors? Error handling: - Detection systems - Recovery procedures - Logging mechanisms - Alert systems - Backup processes - Contingency plans ### What future developments can we expect? Future trends: - Enhanced protocols - Better integration - New features - Advanced capabilities - More tools - Extended support ## Conclusion ## Related Resources Want to learn more about AI agents and automation? Explore these guides: - [Web Scraping 101](/blog/101-scraping) - Master the basics of web scraping - [AI Agent Web Scraping](/blog/ai-agent-webscraping) - Learn about AI-powered scraping - [Mastering ScrapeGraphAI](/blog/mastering-scrapegraphai-endpoint) - Deep dive into our scraping platform - [Building Intelligent Agents](/blog/integrating-scrapegraph-into-intelligent-agents) - Create powerful automation agents - [Pre-AI to Post-AI Scraping](/blog/pre-ai-to-post-ai-scraping) - See how AI has transformed automation - [Structured Output](/blog/structured-output) - Learn about data formatting - [Data Innovation](/blog/data-innovation) - Discover innovative AI solutions - [Full Stack Development](/blog/fullstack-app) - Build complete AI systems - [Web Scraping Legality](/blog/legality-of-web-scraping) - Understand legal considerations